일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 파리 피크닉
- 지베르니 계절 추천
- 42 so_long
- pipex 42
- 와인선별방법
- push swap
- ecole42
- 지베르니 여름
- 포르투갈 여행
- 에꼴42
- get next line
- 42 libft
- push swap 설명
- str함수
- 42
- 서울42
- 지베르니
- 42 pipex
- ft_printf
- gnl
- pipex
- printf
- get_next_line
- 굿노트 스티커
- 지베르니 가을
- 알고리즘 기초
- 와인 고르기
- libft
- so_long
- 이지젯
- Today
- Total
뇌 마음 반반저장소
[42_libft] Part 2 (strmapi, striteri 함수포인터 알아보기) 본문
1-6. ft_strmapi
strmapi란?
Parameters : 매개 변수
s: The string on which to iterate.
s: 반복할 문자열입니다.
f: The function to apply to each character.
f: 각 문자에 적용할 함수입니다.
Return value : 반환 값
The string created from the successive applications of ’f’. Returns NULL if the allocation fails.
'f'의 연속 응용 프로그램에서 생성된 문자열입니다. 할당이 실패하면 NULL을 반환합니다.
External functs. : 외부 기능
malloc
Description : 설명
Applies the function ’f’ to each character of the string ’s’, and passing its index as first argument to create a new string (with malloc(3)) resulting from successive applications of ’f’.
함수 'f'를 문자열 's'의 각 문자에 적용하고 인덱스를 첫 번째 인수로 전달하여 연속적으로 'f'를 적용하여 새 문자열(malloc(3))을 만듭니다.
일단 f가 무엇인지 알아보자. f는 함수를 간단하게 사인처럼 만들어서 변수처럼 사용한다고 생각하면 쉽다.
함수 포인터 알아보기
일단 함수의 구조에 대해서 알아보자.
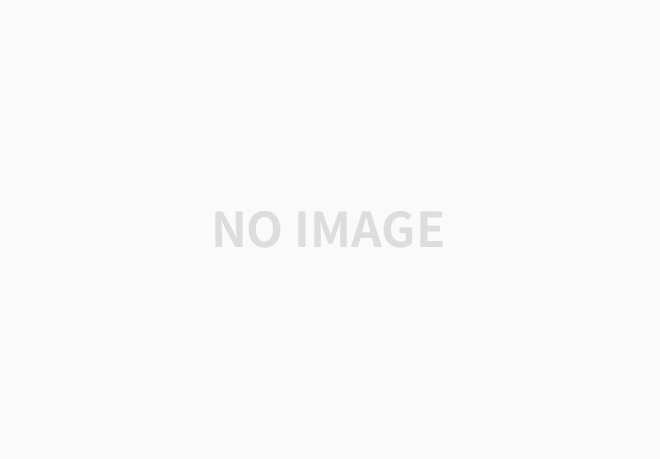
(옛날 옛적 피신을 했을 때 이 구조도 몰라서 헤매었던 나 자신이 생각나면서..)
이 위의 함수를 한 줄로 설명하면 :
'제목'의 함수로 가서 내가 주는 '매개변수'데이터를 이용해서 '반환형'의 값을 돌려주세요.
자 그러면 어떻게 함수 포인터를 사용하는 것일까?
함수 포인터 사용법
함수 포인터의 구조는 이러하다.
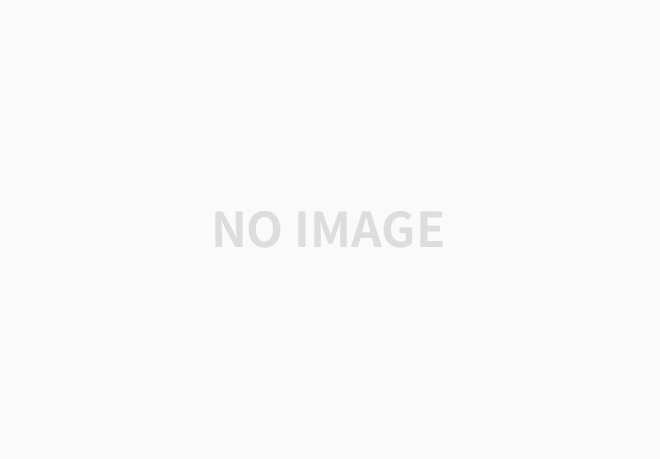
위의 함수와 모양이 굉장히 비슷하다! 쓰임새로 비슷하다. 쉽게 말해서 함수를 통째로 포인터에 저장한다고 생각하면 된다.
이 위의 함수를 한 줄로 설명하면 :
포인터 '제목'에 가면 내가 지정한 함수가 있는데, 거기에 '매개변수'에 입력될 값을 넣고, '반환형'으로 값을 돌려주세요.
아래의 예를 보자.
int ft_plusone(int s)
{
return (s + 1);
}
int main()
{
int (*fp)(int); //함수포인터를 선언해주고
fp = ft_plusone; //함수포인터에 다른 함수로 가라고 손가락질 해준다.
printf("result : %d\n", fp(5));
return(0);
}
결과는!?
$ ./a.out
result : 6
이렇게 잘 나온다. 그리고 여러 함수를 저장하려면 어떻게 해야 할까? 바로 배열을 사용하면 된다! 아래의 예를 보자.
int ft_plusone(int n)
{
return (n + 1);
}
int ft_minusone(int n)
{
return (n - 1);
}
int ft_multitwo(int n)
{
return (n + 2);
}
int ft_divitwo(int n)
{
return (n / 2);
}
int main()
{
int (*fp[4])(int); //함수포인터를 선언해주고
fp[0] = ft_plusone; //배열에 착착 저장한다.
fp[1] = ft_minusone;
fp[2] = ft_multitwo;
fp[3] = ft_divitwo;
printf("result : %d\n", fp[0](6));
printf("result : %d\n", fp[1](6));
printf("result : %d\n", fp[2](6));
printf("result : %d\n", fp[3](6));
return(0);
}
그러면 결과는 이렇게 나온다!
$ ./a.out
result : 7
result : 5
result : 8
result : 3
함수 포인터 응용법 1
함수 포인터는 매개변수로 들어가서 사용될 수 있다. 예를 들면
int ft_plusone(int n)
{
return (n + 1);
}
int ft_result(int a, int b, int (*fp)(int));
{
a = (*fp)(a);
b = (*fp)(b);
return (a + b);
}
int main()
{
int (*fp)(int);
fp = ft_plusone;
printf("result : %d\n", ft_result(5, 10, fp);
return(0);
}
1. 포인터에 plusone함수를 손가락질해두고
2. result함수에서 a는 fp에 있던 plusone함수로 가서 1이 더해진 결과로 돌아오고
b도 1이 더해진 결과로 돌아와서 둘이 합친 결과를 반환 값으로 낸다.
3. 이는 (5 + 1) + (10 + 1) = 17이 나온다!
결과는?
$ ./a.out
result : 17
바로 이것이 우리가 strmapi에서 사용할 형식이다!
함수 선언 원형
char *ft_strmapi(char const *s, char (*f)(unsigned int, char));
!테스트 메인!
각 함수를 s에 적용해 준다. 그리고 문자열 반환.
1. 먼저 적용될 함수가 무엇인지 알 수 없지만, 우리는 함수가 적용된 길이까지 포함해서 길이를 구해야 한다.
먼저 나는 함수 포인트로 지정된 char (*f)(unsigned int, char)를 이해해야 된다고 생각했지만 어디에도 설명이 나오지 않았다.. (이것도 공식인 건가..?) 혹시 아시는 분 댓글로 부탁드려요 ㅠㅠ!
char ft_change(unsigned int i, char word)
{
char new_word;
if (ft_isalpha(word) == 0)
{
return (word);
}
else
{
new_word = word + 32;
}
printf("ft_change %d time : index = %d and %c\n", i, i, new_word);
return (new_word);
}
int main()
{
char *str;
char *result;
str = "HELLO WORLD";
printf("before : %s\n", str);
result = ft_strmapi(str, ft_change);
printf("afte strmapi: %s\n", result);
return (0);
}
!테스트 결과!
$ ./main.out
before : HELLO WORLD
ft_change 0 time : index = 0 and h
ft_change 1 time : index = 1 and e
ft_change 2 time : index = 2 and l
ft_change 3 time : index = 3 and l
ft_change 4 time : index = 4 and o
ft_change 6 time : index = 6 and w
ft_change 7 time : index = 7 and o
ft_change 8 time : index = 8 and r
ft_change 9 time : index = 9 and l
ft_change 10 time : index = 10 and d
afte strmapi: hello world
1-7. ft_striteri
striteri란?
Parameters : 매개 변수
s: The string on which to iterate.
s: 반복할 문자열입니다.
f: The function to apply to each character.
f: 각 문자에 적용할 함수입니다.
Return value : 반환 값
none
External functs. : 외부 기능
none
Description : 설명
Applies the function ’f’ on each character of the string passed as argument, passing its index as first argument. Each character is passed by address to ’f’ to be modified if necessary.
인수로 전달된 문자열의 각 문자에 함수 'f'를 적용하여 인덱스를 첫 번째 인수로 전달합니다. 각 문자는 주소를 통해 'f'로 전달되어 필요한 경우 수정됩니다.
이 함수에 대해서는 많은 정보가 없다. 얼핏 보면 strmapi와 많이 다를 게 없는 함수처럼 보인다. (사실 그렇기도..) 이 친구는 반환 값이 없고 그냥 주소만 찍어주면 된다. 위의 strmapi처럼 하나하나 복사해서 문자열을 내보내 줄 필요가 없다는 점!
함수 선언 원형
void ft_striteri(char *s, void (*f)(unsigned int, char*));
도움을 주고 싶으신 내용이나
틀린 내용이 있다면 댓글로 남겨주시고,
참고하신다면 꼭 출처를 밝혀주세요!
도움이 되었다면 공감 한 번씩 부탁드립니다❤️
'42 > libft' 카테고리의 다른 글
[42_libft] Part 2 (put 함수들과 fd) (0) | 2022.12.15 |
---|---|
[42_libft] Part 2 (itoa) (0) | 2022.12.13 |
[42_libft] Part 2 (split 자세한 설명) (1) | 2022.12.12 |
[42_libft] Part 2 (strtrim) (1) | 2022.12.11 |
[42_libft] Part 2 (substr, strjoin) (0) | 2022.12.11 |